初窥与语法速览
Swift 初窥与语法速览
Comparison
近来
Performance
编译器优化
注释与换行
注释
请将你的代码中的非执行文本注释成提示或者笔记以方便你将来阅读。
// 这是一个注释
你也可以进行多行注释,其起始标记为单个正斜杠后跟随一个星号
/* 这是一个, 多行注释 */
与
/* 这是第一个多行注释的开头 /* 这是第二个被嵌套的多行注释 */ 这是第一个多行注释的结尾 */
通过运用嵌套多行注释,你可以快速方便的注释掉一大段代码,即使这段代码之中已经含有了多行注释块。
语法要点
! VS ?
在
It may be easiest to remember the pattern for these operators in Swift as: ! implies “this might trap,” while ?indicates “this might be nil.”
就是
Quick Start
Objective-C 混合编程
参考资料
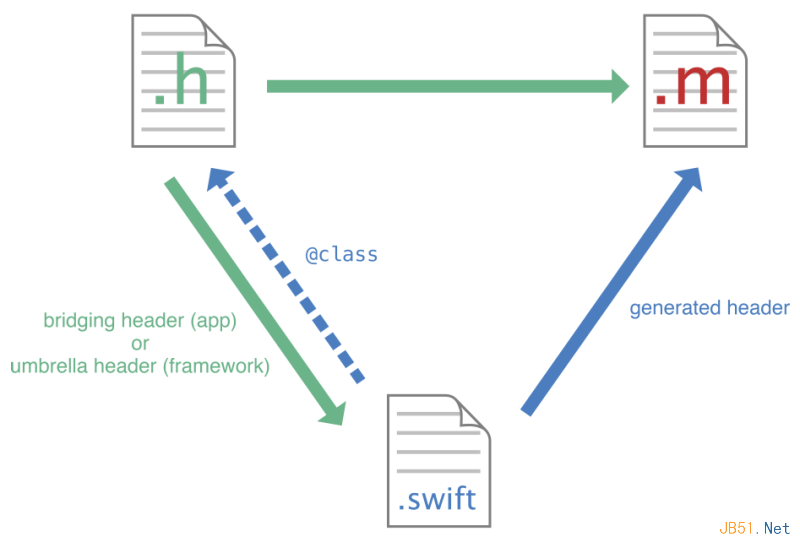
Swift 类引用Objective-C 文件
因为
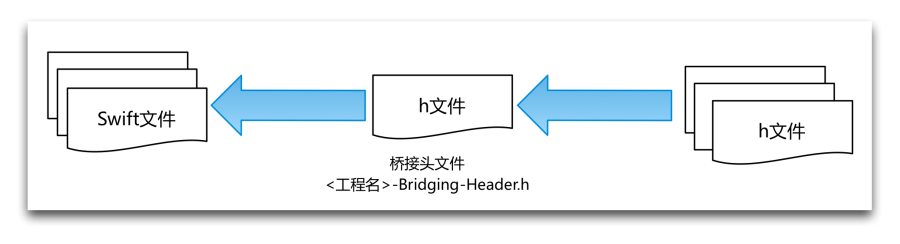
OJC 类如下:
//
// ObjcFunc.h
//
# import <Foundation/Foundation.h>
@interface ObjcFunc : NSObject
-(NSString*)sayHello:(NSString*)greeting withName: (NSString*)name;
@end
//
// ObjcFunc.m
//
# import "ObjcFunc.h"
# import "CombinedProgram-Swift.h"
@implementation ObjcFunc
- (NSString*)sayHello:(NSString*)greeting withName: (NSString*)name{
NSString *string = [NSString stringWithFormat:@"Hi,%@ %@.",name,greeting];
return string;
}
@end
Swift 类中调用
import Foundation
@objc class SwiftFunc: NSObject {
func sayHello() -> Void {
var obj : ObjcFunc = ObjcFunc()
println(obj.sayHello("Hello", withName: "Swift"));
return
}
}
Objective-C 类引用Swift 文件
#import "{ModuleName}-swift.h"
SwiftFunc* obj = [[SwiftFunc alloc] init];
[obj sayHello];
有时候会发现
Product Module Name : myproject
Defines Module : YES
Embedded Content Contains Swift : YES
Install Objective-C Compatibility Header : YES
sObjective-C Bridging Header : $(SRCROOT)/Sources/SwiftBridging.h
要保证你的
流程控制
分支选择
if
if 1+1 == 2 {
println("The math checks out")
}
if-let
可以用
var conditionalString : String? = "a string"
if let theString = conditionalString {
println("The string is '\(theString)'")
}
else {
println("The string is nil")
}
// 输出 "The string is 'a string'"
同时
var conditionalString : String? = "a string"
if let theString = conditionalString where theString == "a"{
print("The string is '\(theString)'")
} else {
print("The string is nil")
}
guard: 保镖模式
与
func fooGuard(x: Int?) {
guard let x = x where x > 0 else {
// 变量不符合条件判断时,执行下面代码
return
}
// 使用x
x.description
}
是对你所期望的条件做检查,而非不符合你期望的。又是和
func fooNonOptionalGood(x: Int) {
guard x > 0 else {
// 变量不符合条件判断时,执行下面代码
return
}
// 使用x
}
func fooNonOptionalBad(x: Int) {
if x <= 0 {
// 变量不符合条件判断时,执行下面代码
return
}
// 使用x
}
循环
for
for-in
let loopingArray = [1,2,3,4,5]
var loopSum = 0
for number in loopingArray {
loopSum += number
}
loopSum // = 15
var firstCounter = 0
for index in 1 ..< 10 {
firstCounter++
}
// 循环9次
var secondCounter = 0
for index in 1 ... 10 { // 注意是三个句点,不是两个
secondCounter++
}
// 循环10次
var sum = 0
for var i = 0; i < 3; i++ {
sum += 1
}
sum // = 3
while
var countDown = 5
while countDown > 0 {
countDown--
}
countDown // = 0
var countUP = 0
do {
countUp++
} while countUp < 5
countUp // = 5
defer: 代码块延迟执行
可以在同一个作用域中指定多个
例如下面的代码:
openFile()
defer {
// defer block 1
closeFile()
}
startPortListener(42)
defer {
// defer block 2
stopPortListener(42)
}
这段代码在作用域结束的时候,第二个
异常处理
异常捕获
try?
可以使用
func someThrowingFunction() throws -> Int {
// ...
}
let x = try? someThrowingFunction()
let y: Int?
do {
y = try someThrowingFunction()
} catch {
y = nil
}
如果
func fetchData() -> Data? {
if let data = try? fetchDataFromDisk() { return data }
if let data = try? fetchDataFromServer() { return data }
return nil
}
譬如以
func parse(fromData data: NSData) throws -> TodoList {
do {
guard let jsonDict = try NSJSONSerialization.JSONObjectWithData(data, options: .AllowFragments) as? [String : AnyObject],
// todoListDict is now moved up here
todoListDict = jsonDict["todos"] as? [[String : AnyObject]] else {
throw Error.InvalidJSON
}
let todoItems = todoListDict.flatMap { TodoItemParser().parse(fromData: $0) }
return TodoList(items: todoItems)
} catch {
throw Error.InvalidJSON
}
struct TodoListParser {
enum Error: ErrorType {
case InvalidJSON
}
func parse(fromData data: NSData) throws -> TodoList {
guard let jsonDict = try? NSJSONSerialization.JSONObjectWithData(data, options: .AllowFragments) as? [String : AnyObject],
// Notice the extra question mark here!
todoListDict = jsonDict?["todos"] as? [[String : AnyObject]] else {
throw Error.InvalidJSON
}
let todoItems = todoListDict.flatMap { TodoItemParser().parse(fromData: $0) }
return TodoList(items: todoItems)
}
}